Tokenizer Integration
Seamless PCI-compliant integration of creditcard payments
node.js seamless quickstart
The quickstart guide is available here
A complete seamless integration example is available here
With the Tokenizer Integration the merchant requests a payment input form from mPAY24 to embed it in his payment page. The mPAY24 system generates the input form for the customer's payment credentials and returns the URL as well as a token to the merchant. The merchant embeds this URL in an iFrame on his payment page. The customer stays on the merchant's website and enters the payment credentials in the pre-generated input form. The entered data are automatically submitted to the mPAY24 server and stored. After completing the order, the merchant has to use the token, which he received upon requesting the payment form, to initiate a payment with the stored data.
The merchant does not gain access to the payment credentials entered by the customer in the input form. Only limited information about the payment data are available to the merchant (e.g. an obscured credit card number).
Supported payment methods
Currently only CreditCards and MAESTRO are supported. For other payment methods use the Backend2Backend Integration.
The Tokenizer Integration uses the CreatePaymentToken
and AcceptPayment
operation. The resulting input form of the CreatePaymentToken
call can be styled via a stored styling.
mPAY24 strongly recommends to embed the payment form (tokenizerUrl) only on HTTPS secured websites!
Integration steps
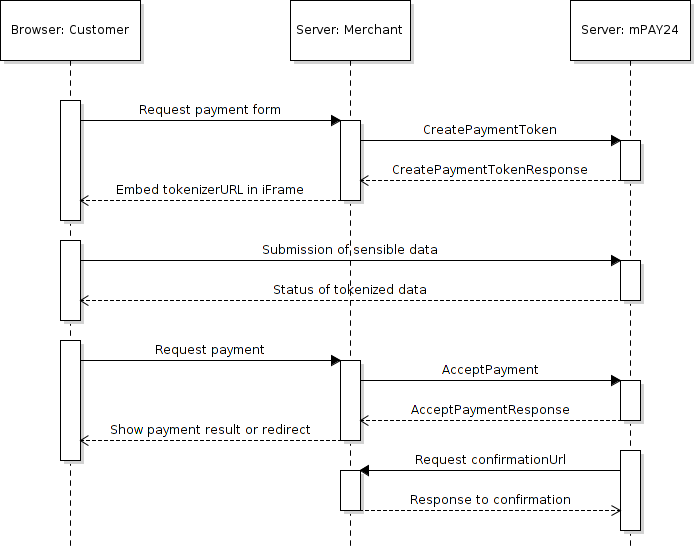
[Workflow diagram of the Tokenizer Integration variant
-
The customer requests a payment page to complete his order.
-
The merchant sends a
CreatePaymentToken
call including details (e.g. for styling specifications) to mPAY24.
<SOAP-ENV:Envelope
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:etp="https://www.mpay24.com/soap/etp/1.5/ETP.wsdl">
<SOAP-ENV:Header/>
<SOAP-ENV:Body>
<etp:CreatePaymentToken>
<merchantID>90000</merchantID>
<pType>CC</pType>
<templateSet>DEFAULT</templateSet>
<style>DEFAULT</style>
<language>EN</language>
</etp:CreatePaymentToken>
</soap:Body>
</soap:Envelope>
<SOAP-ENV:Envelope
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:etp="https://www.mpay24.com/soap/etp/1.5/ETP.wsdl">
<SOAP-ENV:Header/>
<SOAP-ENV:Body>
<etp:CreatePaymentTokenResponse>
<status>OK</status>
<returnCode>REDIRECT</returnCode>
<token>TBXAkjhGf[...]</token>
<apiKey>e23b9ad6[...]</apiKey>
<location>https://test.mpay24.com/app/bin/tokenizer/fragment/e23b9[...]</location>
</etp:CreatePaymentTokenResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
mpay24.createPaymentToken({
pType: 'CC',
templateSet: 'DEFAULT',
style: 'DEFAULT',
}).then(data => {
data.location //url of the tokenizer
}).catch(err => {
console.error(err);
});
- mPAY24 returns the
CreatePaymentTokenResponse
including areturnCode = REDIRECT
and alocation
value (ifstatus = OK
). - The merchant embeds the tokenizerURL of the
location
element in an iFrame on the merchant payment page. - The customer enters the sensible payment information in the form (e.g. credit card number).
The embedded payment form does only store the data for a temporary time and mPAY24 does not automatically initiate a payment.
Demo
Card Number: 4444 3333 2222 1111
Valid thru: 05/25
CVC / CVN: 123
- The iFrame communicates with the merchants page to inform when the payment can be sent. A detailed description about this process can be found in the iFrame Communication chapter.
- The customer confirms the payment (e.g. by pressing a
Pay
button). - The merchant uses the payment token to initiate the payment with a
AcceptPayment
request.
<SOAP-ENV:Envelope
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:etp="https://www.mpay24.com/soap/etp/1.5/ETP.wsdl"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<SOAP-ENV:Header/>
<SOAP-ENV:Body>
<etp:AcceptPayment>
<merchantID>90000</merchantID>
<tid>123</tid>
<pType>TOKEN</pType>
<payment xsi:type="etp:PaymentTOKEN">
<amount>100</amount>
<currency>EUR</currency>
<token>TBXAkjhGfa[...]</token>
</payment>
<customerName>John Doe</customerName>
<order>
<description>Example payment</description>
</order>
<successURL>http://www.hotelmuster.at/succ.php</successURL>
<errorURL>http://www.hotelmuster.at/err.php</errorURL>
<confirmationURL>http://www.hotelmuster.at/conf.php</confirmationURL>
</etp:AcceptPayment>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
mpay24.acceptPayment({
tid: '123',
pType: 'TOKEN',
payment: {
amount: 100,
currency: 'EUR',
token: 'TBXAkjhGfa[...]',
},
successURL: 'http://www.hotelmuster.at/succ.php',
errorURL: 'http://www.hotelmuster.at/err.php',
confirmationURL: 'http://www.hotelmuster.at/conf.php',
}).then(result => {
result.status; // OK
result.returnCode; // OK or REDIRECT (3DS)
result.location; // only set if REDIRECT
console.log(result);
}).catch(err => {
console.error(err);
});
<SOAP-ENV:Envelope
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:etp="https://www.mpay24.com/soap/etp/1.5/ETP.wsdl"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<SOAP-ENV:Header/>
<SOAP-ENV:Body>
<etp:AcceptPayment>
<merchantID>90000</merchantID>
<tid>123</tid>
<pType>TOKEN</pType>
<payment xsi:type="etp:PaymentTOKEN">
<amount>100</amount>
<currency>EUR</currency>
<token>TBXAkjhGfa[...]</token>
<useProfile>true</useProfile>
</payment>
<customerName>John Doe</customerName>
<customerID>123456789</customerID>
<order>
<description>Example payment</description>
</order>
<successURL>http://www.hotelmuster.at/succ.php</successURL>
<errorURL>http://www.hotelmuster.at/err.php</errorURL>
<confirmationURL>http://www.hotelmuster.at/conf.php</confirmationURL>
</etp:AcceptPayment>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
- mPAY24 returns the
AcceptPaymentResponse
with information about the transaction result.
PSD2 and Strong Customer Authentication
Due to the upcoming changes (3DS 2.X) mandatory personal data are:
Cardholder name and e-mail-address.However we recommend providing as much customer data as possible.
It is possible that the
AcceptPaymentResponse
returns alocation
and the customer has to be redirected to a third party website. See chapter Processing a redirection payment of the Backend2Backend Integration for a detailed workflow of handling redirections with theAcceptPaymentResponse
.
-
The merchant informs the customer about the payment/transaction.
-
mPAY24 communicates the payment result via push using the
confirmationURL
(see chapter Payment notification for more information). (Confirmation Interface). Note that this step could occur before mPAY24 returned theAcceptPaymentResponse
.
http://www.hotelmuster.com/conf.php?OPERATION=CONFIRMATION&TID=4002451&
STATUS=RESERVED&PRICE=1000&CURRENCY=EUR&P_TYPE=CC&BRAND=VISA&MPAYTID=1683862&
USER_FIELD=&ORDERDESC=Example+payment&CUSTOMER=John+Doe&CUSTOMER_EMAIL=&LANGUAGE=DE&
CUSTOMER_ID=&PROFILE_STATUS=IGNORED&FILTER_STATUS=OK&APPR_CODE=%2Dtest%2D
- The merchant confirms the receipt of the transaction notification with either
OK
orERROR
(status depends if the confirmation could successfully update the merchant' system).
In
PHP
this could be achieved byecho 'OK'
iFrame Communication
It is recommended that the merchant implements a communication via JavaScript between the iFrame of mPAY24 and his payment site. Due to this communication, the merchant is informed if the brand or other payment information are changed.
window.onload = new function() {
window.addEventListener("message", checkValid, false);
function checkValid(form) {
var data = JSON.parse(form.data);
// if true unlock button for payment
if (data.valid === "true") {
//ex.: document.getElementById("paybutton").disabled=false;
}
if(data.brandChange){
console.log(data.brandChange);
}
}
}
The following events can be obtained by the iFrame via JavaScript:
- Brand change
Upon change, the detected brand is returned. If the brand is not recognized or the card number has been deleted, the value none
is returned.
Possible values: `amex`, `jcb`, `diners`, `discover`, `mastercard`, `visa`, `none`
{
brandChange: "mastercard"
}
- Input of a form field
After a field (identifier, expiry or cvc) is entered, the data is submitted securely to the mPAY24 system. After storing the data we will trigger an event with the stored data and the validity.
The valid
element, which defines if the payment data are correctly entered and complete. Only if valid: 'true'
, the token can be used for processing the payment.
{
valid: "false",
response: {
brand:"VISA",
identifier:"************1111",
expiry:"",
cvc:""
}
}
{
valid: "true",
response: {
brand:"VISA",
identifier:"************1111",
expiry:"2005",
cvc:"***"
}
}
- Onfocus & Onblur events
These can be ignored in the normal tokenizer integration (if you don't use each field separately)
This events should provide you the information which field is the active one. This is done because we don't provide any "active" input field styling to offer you more flexibility for the single fields.
After receiving an "onfocus" event, you could add a border to the iframe to indicate that he is currently focusing this input field
The following example shows you how to add a border to the current active field.
{
event: "onfocus",
field: "identifier"
}
window.onload = new function() {
window.addEventListener("message", checkValid, false);
function checkValid(form) {
var data = JSON.parse(form.data);
if (data.event === "onfocus") {
document.getElementById(data.field + "Frame").style.border = "1px solid red";
} else if (data.event === "onblur") {
document.getElementById(data.field + "Frame").style.border = "none";
}
}
}
Get raw input fields
For even more flexibility you can get each input field without labels, size or styling.
This can be done by adding /identifier
, /expiry
or /cvc
to the location.
Example: https://test.mpay24.com/app/bin/tokenizer/fragment/[...]/identifier
This returns just one input field without border, outline or other styling.
There are following GET parameter you can append to customize these inputs
Field(s) | Parameter | Description |
---|---|---|
/identifier /expiry /cvc | ?placeholder=custom | You can override or set a placeholder for each input field |
/identifier | ?fontsize=20 | You can override the font size in px |
/identifier | ?hidebrand | If you don't want to show the current brand ( |
Demo Raw
Input form styling
A styling for the input form can be deposited for a merchant's account to be used during the CreatePaymentToken
call. To create the styling the mPAY24 Designer should be used, which is located at https://test.mpay24.com/web/designer.
The generated XML needs to be mailed to the mPAY24 Support Team.
mPAY24 form fields session and idle timeouts
The form fields created by the CreatePaymentToken
is configured for a total session timeout of 30 minutes. The session idle timeout is set to 15 minutes. If one of these timeouts is exceeded, the session is terminated and the payment should be restarted. After the session is terminated the stored data are deleted and can not be used via an AcceptPayment
call.
Updated 27 days ago